You can interact with this notebook online: Launch interactive version
Generating Data Exploration Widgets¶
A demonstration of how to generate TARDIS widgets that allows you to explore simulation data within Jupyter Notebook with ease!
This notebook is a quickstart tutorial, but more details on each widget (and its features) is given in the Using TARDIS Widgets section of the documentation.
First create and run a simulation that we can use to generate widgets (more details about running simulation in Quickstart section):
[1]:
from tardis import run_tardis
from tardis.io.atom_data.util import download_atom_data
# We download the atomic data needed to run the simulation
download_atom_data('kurucz_cd23_chianti_H_He')
sim = run_tardis('tardis_example.yml', virtual_packet_logging=True)
/usr/share/miniconda3/envs/tardis/lib/python3.7/importlib/_bootstrap.py:219: QAWarning: pyne.data is not yet QA compliant.
return f(*args, **kwds)
[py.warnings ][WARNING] /usr/share/miniconda3/envs/tardis/lib/python3.7/site-packages/traitlets/traitlets.py:3050: FutureWarning: --rc={'figure.dpi': 96} for dict-traits is deprecated in traitlets 5.0. You can pass --rc <key=value> ... multiple times to add items to a dict.
FutureWarning,
(warnings.py:110)
[py.warnings ][WARNING] /usr/share/miniconda3/envs/tardis/lib/python3.7/site-packages/tardis-2021.10.14.0.dev89+gefebacd9-py3.7.egg/tardis/montecarlo/montecarlo_numba/base.py:102: VisibleDeprecationWarning:
Creating an ndarray from ragged nested sequences (which is a list-or-tuple of lists-or-tuples-or ndarrays with different lengths or shapes) is deprecated. If you meant to do this, you must specify 'dtype=object' when creating the ndarray
(warnings.py:110)
[py.warnings ][WARNING] /usr/share/miniconda3/envs/tardis/lib/python3.7/site-packages/tardis-2021.10.14.0.dev89+gefebacd9-py3.7.egg/tardis/montecarlo/montecarlo_numba/base.py:105: VisibleDeprecationWarning:
Creating an ndarray from ragged nested sequences (which is a list-or-tuple of lists-or-tuples-or ndarrays with different lengths or shapes) is deprecated. If you meant to do this, you must specify 'dtype=object' when creating the ndarray
(warnings.py:110)
[py.warnings ][WARNING] /usr/share/miniconda3/envs/tardis/lib/python3.7/site-packages/tardis-2021.10.14.0.dev89+gefebacd9-py3.7.egg/tardis/montecarlo/montecarlo_numba/base.py:108: VisibleDeprecationWarning:
Creating an ndarray from ragged nested sequences (which is a list-or-tuple of lists-or-tuples-or ndarrays with different lengths or shapes) is deprecated. If you meant to do this, you must specify 'dtype=object' when creating the ndarray
(warnings.py:110)
[py.warnings ][WARNING] /usr/share/miniconda3/envs/tardis/lib/python3.7/site-packages/tardis-2021.10.14.0.dev89+gefebacd9-py3.7.egg/tardis/montecarlo/montecarlo_numba/base.py:111: VisibleDeprecationWarning:
Creating an ndarray from ragged nested sequences (which is a list-or-tuple of lists-or-tuples-or ndarrays with different lengths or shapes) is deprecated. If you meant to do this, you must specify 'dtype=object' when creating the ndarray
(warnings.py:110)
[py.warnings ][WARNING] /usr/share/miniconda3/envs/tardis/lib/python3.7/site-packages/tardis-2021.10.14.0.dev89+gefebacd9-py3.7.egg/tardis/montecarlo/montecarlo_numba/base.py:114: VisibleDeprecationWarning:
Creating an ndarray from ragged nested sequences (which is a list-or-tuple of lists-or-tuples-or ndarrays with different lengths or shapes) is deprecated. If you meant to do this, you must specify 'dtype=object' when creating the ndarray
(warnings.py:110)
[py.warnings ][WARNING] /usr/share/miniconda3/envs/tardis/lib/python3.7/site-packages/tardis-2021.10.14.0.dev89+gefebacd9-py3.7.egg/tardis/montecarlo/montecarlo_numba/base.py:117: VisibleDeprecationWarning:
Creating an ndarray from ragged nested sequences (which is a list-or-tuple of lists-or-tuples-or ndarrays with different lengths or shapes) is deprecated. If you meant to do this, you must specify 'dtype=object' when creating the ndarray
(warnings.py:110)
[py.warnings ][WARNING] /usr/share/miniconda3/envs/tardis/lib/python3.7/site-packages/tardis-2021.10.14.0.dev89+gefebacd9-py3.7.egg/tardis/montecarlo/montecarlo_numba/base.py:120: VisibleDeprecationWarning:
Creating an ndarray from ragged nested sequences (which is a list-or-tuple of lists-or-tuples-or ndarrays with different lengths or shapes) is deprecated. If you meant to do this, you must specify 'dtype=object' when creating the ndarray
(warnings.py:110)
[py.warnings ][WARNING] /usr/share/miniconda3/envs/tardis/lib/python3.7/site-packages/tardis-2021.10.14.0.dev89+gefebacd9-py3.7.egg/tardis/montecarlo/montecarlo_numba/base.py:123: VisibleDeprecationWarning:
Creating an ndarray from ragged nested sequences (which is a list-or-tuple of lists-or-tuples-or ndarrays with different lengths or shapes) is deprecated. If you meant to do this, you must specify 'dtype=object' when creating the ndarray
(warnings.py:110)
Now, import functions & class to create widgets from visualization
subpackage:
[2]:
from tardis.visualization import (
shell_info_from_simulation,
shell_info_from_hdf,
LineInfoWidget,
)
Shell Info Widget¶
This widget allows you to explore chemical abundances of each shell - all the way from elements to ions to levels - by just clicking on the rows you want to explore!
There are two ways in which you can generate the widget:
Using a Simulation object¶
We will use the simulation object we created in the beginning, sim
to generate shell info widget. Then simply display it to start using.
[3]:
shell_info_widget = shell_info_from_simulation(sim)
shell_info_widget.display()
You can interact with the widget produced in output above (which won’t be visible if you’re viewing this notebook in our docs as an html page) like this:
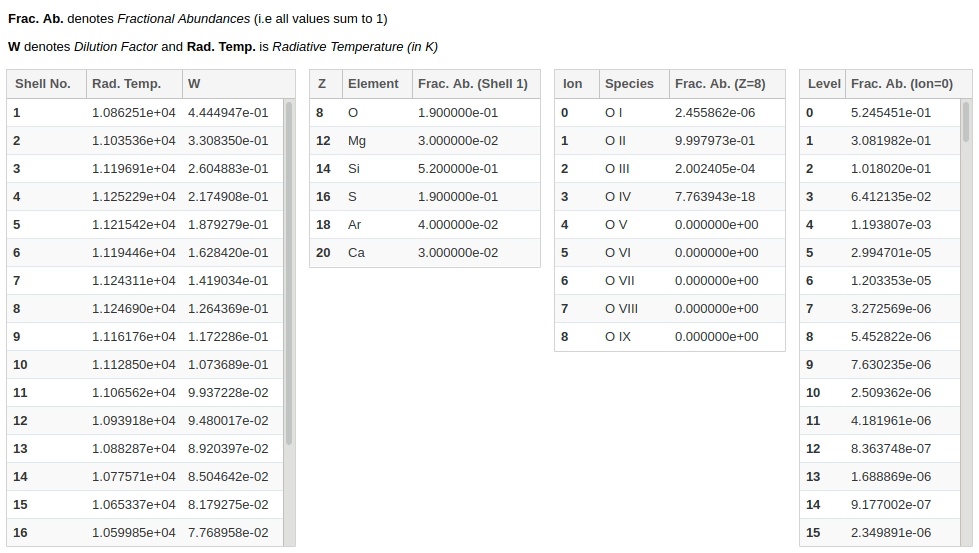
Use the button at the top of this page to run the notebook in interactively to use the widgets!
Using a saved simulation (HDF file)¶
Alternatively, if you have a TARDIS simulation model saved on your disk as an HDF file, you can also use it to generate the shell info widget.
[4]:
# shell_info_widget = shell_info_from_hdf('demo.hdf')
# shell_info_widget.display()
Line Info Widget¶
This widget lets you explore the atomic lines responsible for producing features in the simulated spectrum.
You can select any wavelength range in the spectrum interactively to display a table giving the fraction of packets that experienced their last interaction with each species. Using toggle buttons, you can specify whether to filter the selected range by the emitted or absorbed wavelengths of packets. Clicking on a row in the species table, shows packet counts for each last line interaction of the selected species, which can be grouped in several ways.
To generate line info widget, we will again use the simulation object sim
and then display the widget:
[5]:
line_info_widget = LineInfoWidget.from_simulation(sim)
line_info_widget.display()
You can interact with this widget (which again won’t be visible if you’re viewing this notebook in our docs as an html page) like this:
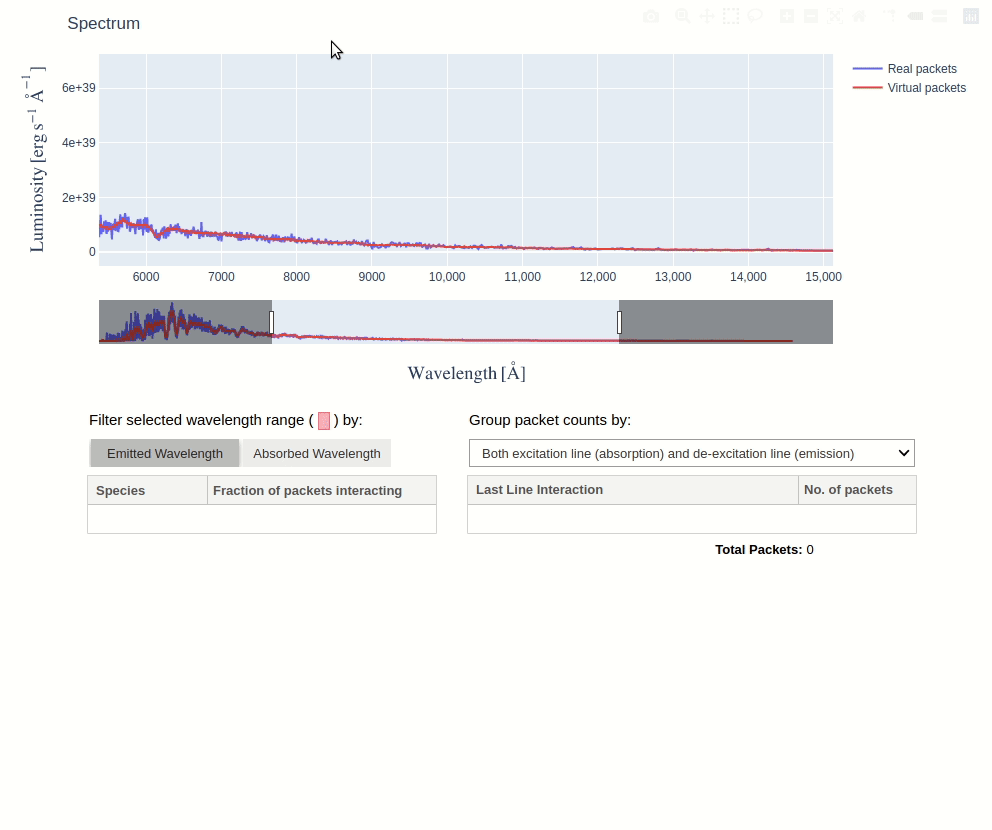
Note
The virtual packet logging capability must be active in order to produce virtual packets’ spectrum in Line Info Widget
. Thus, make sure to set virtual_packet_logging: True
in your configuration file. It should be added under virtual
property of spectrum
property, as described in configuration schema.